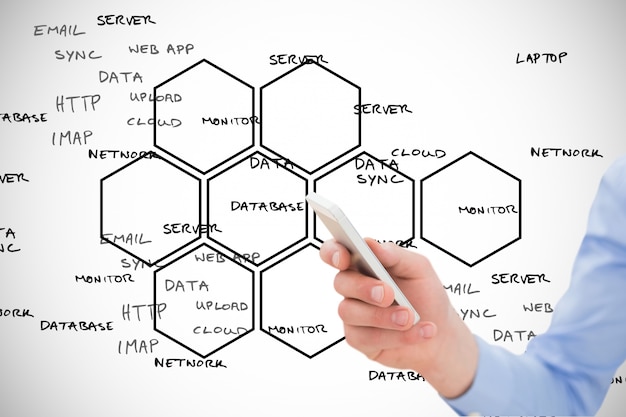
Understanding Complex Conditional Structures
Conditional structures are an essential part of programming that allow us to make decisions based on certain conditions. They provide a way to control the flow of a program, executing different blocks of code based on whether a condition is true or false. While simple conditional statements like if and else are commonly used, there are situations where more complex conditional structures are required.
What are Complex Conditional Structures?
Complex conditional structures, also known as nested conditionals, are combinations of multiple conditional statements within one another. They allow us to create more sophisticated decision-making processes by evaluating multiple conditions and executing different blocks of code accordingly.
By nesting conditional statements, we can create complex logical evaluations that cover a wide range of scenarios. These structures often involve the use of logical operators, such as AND (&&) and OR (||), to combine multiple conditions and determine the final outcome.
Example of Complex Conditional Structures
Let’s consider an example to understand complex conditional structures better. Suppose we have a grading system where students’ final grades are determined based on their individual scores in three exams: Math, Science, and English. The grading system follows the following rules:
Subject | Minimum Score |
---|---|
Math | 70 |
Science | 60 |
English | 75 |
In this scenario, a student’s final grade is determined as follows:
- If the student scores below the minimum required in any one subject, they fail.
- If the student scores above the minimum required in all three subjects, they pass.
- If the student scores above the minimum required in at least two subjects, they achieve a merit grade.
- If the student scores above the minimum required in only one subject, they achieve a standard grade.
Using complex conditional structures, we can implement this grading system in our program. Here’s an example in pseudocode:
if (mathScore < 70 || scienceScore < 60 || englishScore < 75) { grade = "Fail"; } else if (mathScore > 70 && scienceScore > 60 && englishScore > 75) { grade = "Pass"; } else if (mathScore > 70 && scienceScore > 60 || mathScore > 70 && englishScore > 75 || scienceScore > 60 && englishScore > 75) { grade = "Merit"; } else { grade = "Standard"; }
In the above example, we evaluate each condition separately and determine the appropriate grade based on the combination of scores.
Practice Exercises
Now that you understand complex conditional structures, here are some practice exercises to test your knowledge. Try to solve these exercises on your own before checking the answers below:
Exercise 1:
Write a program that determines whether a given year is a leap year. A leap year is a year that is divisible by 4 but not divisible by 100, except if it is divisible by 400. The program should output “Leap year” if the given year is a leap year, and “Not a leap year” otherwise.
Exercise 2:
Write a program that calculates the cost of shipping based on the weight and destination of a package. The shipping rates are as follows:
Destination | Rate per pound |
---|---|
Local | $1.50 |
Domestic | $2.50 |
International | $5.00 |
The program should ask the user for the weight and destination of the package, and then calculate and display the total shipping cost.
Exercise Answers
Exercise 1:
if (year % 4 == 0) { if (year % 100 == 0) { if (year % 400 == 0) { output "Leap year"; } else { output "Not a leap year"; } } else { output "Leap year"; } } else { output "Not a leap year"; }
Exercise 2:
input weight input destination if (destination == "Local") { cost = weight * 1.50; } else if (destination == "Domestic") { cost = weight * 2.50; } else if (destination == "International") { cost = weight * 5.00; } output "Total shipping cost: $" + cost;
Complex conditional structures provide us with the flexibility to handle various scenarios and make decisions based on multiple conditions. By understanding and practicing these structures, you can become more proficient in programming and problem-solving. Keep exploring and honing your skills to become a confident developer!